How do you set up a React or Angular application from scratch? In this tutorial, I will show you how to set up the 'mood' application, starting from a clean slate.
Download RunMe.app.Spoof your Serial Number (Clover and OpenCore) -Kextstat -Pmset -Xcpm -ACPI tables -Kextload -AudioDeviceName -VDADecoder Checker -ig-platform-id -Ioreg -Bootargs -Kernel Version -Audio Codecs -Kernel -System Version -Full Kextstat -Cpu -IOBuiltin -Boot. Download Age Face - Make me OLD App on Windows PC with LDPlayer. Use Age Face - Make me OLD App easily on PC. Enjoy Age Face - Make me OLD with larger screen and better picture quality. Best way to run multiple WhatsApp, Games, any App on one Phone. Easiest Method.to run two accounts in one Android Phone. Android Dual Apps. In👏 this👏 house👏 we👏 respect👏 all👏 ships. Do you want to run iOS apps on Android Phone? Here I have collected the list of iOS Emulators for Android that helps you to run iOS apps on your device.
Use create-react-app, pass in your application name and use the typescript template: npx create-react-app mood-react --template typescript
.
Use the Angular CLI to create a new application and supply the name: npx -p @angular/cli ng new mood-ng
.
Creating an Application
You can create both Angular and React applications with a single call to a command line tool. While Angular comes with the Angular CLI, and it is highly recommended that you use it, there are many different ways to create a React application. Theoretically, you do not need a toolchain at all for React. But in this tutorial, I will show you how to use create-react-app.
Creating a React Application
The easiest way to create a single-page application ('SPA') using React is to use create-react-app:
A few things are happening here:
- I use
npx
to run create-react-app directly. I could have also installed it globally, but there is no real reason to do that. - The next parameter, 'mood-react' is the name of the application. create-react-app creates a folder with this name and initializes the React application inside.
- '--template typescript' tells the command line tool that I want to use typescript in my React application.
create-react-app adds all dependencies to the newly created project. It also provides scripts to run and test your application and to create a production build.
Creating an Angular Application
Angular comes with a command line interface, which is Angular CLI. You will use this to create your application and for many different tasks later on. To create an application, run
In most cases, you should also supply the --strict parameter to enable strict injection parameter checking and strict template type checking.
In the angular documentation, you will read that you should install the angular command line tool globally (instead of using npx
). But since npx
is a thing, I do not want to install npm scripts globally anymore. And when you're creating the project, the CLI will install itself inside your application, too, so you can run it from there.
The command I just ran with npx
was ng new mood-ng
, where ng is the Angular CLI, new is the command to run, and mood-ng is the name of the new application I wanted to create. The Angular CLI also provides some scripts to run, test and build the application:
It also provides a script to run the CLI itself:
Project Structure
Both tools, create-react-app and the Angular CLI, scaffold a project structure for you. Both tools create support for unit testing and some example unit tests. The Angular CLI also creates end-to-end tests. Both applications provide a development server that will automatically reload the browser when you save a file.
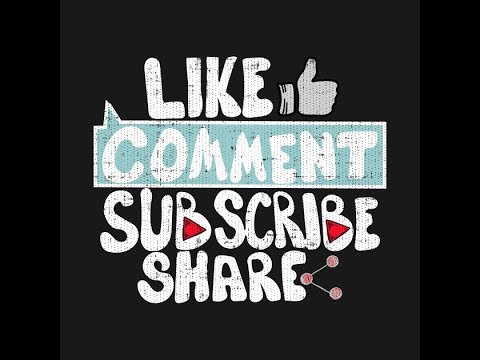
Project Structure in React
create-react-app creates a scaffold for your application. The initial directory structure looks like this, but with more files in each directory (denoted by ...
):
You can place your static assets in the public
folder, while dynamic source code resides in src
. In the project structure created by the command line tool, the unit tests reside in the same folder structure as the components themselves, since they belong to the component.
All typescript files shown above use the extension .tsx
. This tells the toolchain that the file can contain JSX code, which is code that looks like HTML but compiles to JavaScript. I will write about that in a later section. For now, remember to name your files .tsx
if they contain React components or other code that uses JSX and .ts
otherwise (services, utility functions,...).
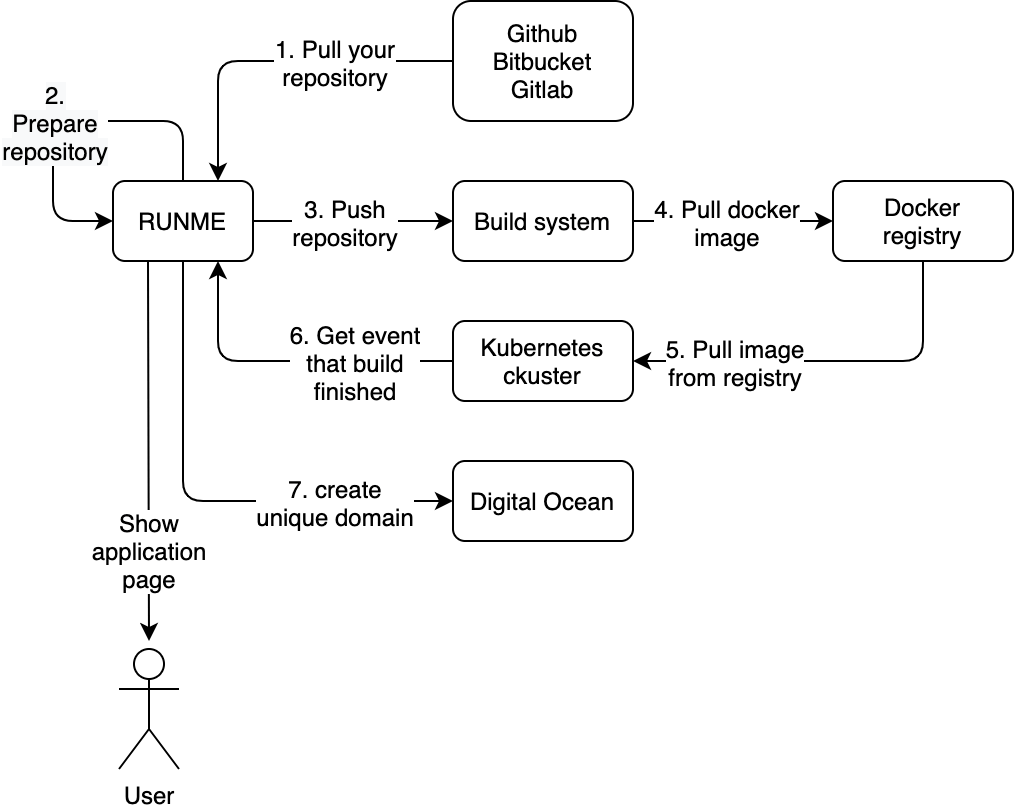
src/index.tsx
contains the code to bootstrap the application:
This code looks for a DOM element with the ID root and renders the App component inside it (actually, inside another component called React.StrictMode, but you can ignore that for now).
There is one component called App in App.tsx
and it comes with its own CSS (App.css
) and unit test (App.test.tsx
). When you start the development server with npm start
, your browser will navigate to http://localhost:3000/ and show:
Project Structure in Angular

The Angular CLI also scaffolds a project for you. The basic project structure looks like this:
The Angular CLI creates more files and directories because Angular includes some features by default, whereas in React, you would use additional libraries.
All sources, including static assets, reside in the src
folder. The directory called e2e
contains end-to-end tests written using Protractor. In the project structure created by the command line tool, the unit tests reside in the same folder structure as the components themselves, since they belong to the component.
src/main.ts
contains the code to start up the Angular application:
This code bootstraps the AppModule, which is defined in src/app/app.module.ts
. It registers the app's only component, AppComponent, defined in src/app/app.component.ts
, which provides the selector (think 'custom HTML element') app-root
.
So, after bootstrapping, the line <app-root></app-root>
in src/index.html
will display exactly that component:
After starting the development server with npm start
, you can navigate your browser to http://localhost:4200/, and then you will see:
Styles and Assets
Both the create-react-app and the Angular CLI configure folders for static assets, like images, stylesheets and more. Both React and Angular also allow per-component CSS files to encapsulate the styles of a component, but the approaches are slightly different here...
Styles and Assets in React
Add the static assets of your application in the public
folder:
Here, you can add images, global CSS files (if you need any) and third-party CSS, fonts, etc. It is completely up to you how you organize the files in this public folder.
You can also add per-component CSS files (and even other assets). Look at the src/App.tsx
component that the command line tool has created for you:
Two interesting things are happening here:
The component imports ./App.css
, but this is not a 'normal' JavaScript import. The command line tool did configure webpack to extend the concept of imports to loading CSS. In the production build of the application, all those component-specific styles will be collected into a single, minified CSS file.
This approach to styling components is convenient, but it is not required for a React application to use it. I will show you other ways to style your components later in this guide.

The component also imports logo
from an SVG image. This functionality is also provided by webpack. By including the line import logo from './logo.svg';
in the component, we tell webpack that this SVG image should be a part of the final bundle. The value returned in logo
is a path to that file. The component can use it to reference this file—in our case, as the src
of an img
.
Styles and Assets in Angular
Add the static assets of your application in the src/assets
folder (for more information, visit the documentation of the generated file structure):
Runme App Windows
You can, again, put all kinds of static assets here: Global CSS, images, third-party fonts, etc. How you organize the content in this folder is completely up to you.
Angular components usually consist of multiple files: A typescript class that contains the application logic, a typescript file that contains the unit-tests for the component, a template HTML file, and often also a CSS file that contains the per-component styles:
So, angular also supports encapsulating the style of a component directly in the component itself. The component's code references the CSS file from the component's decorator:
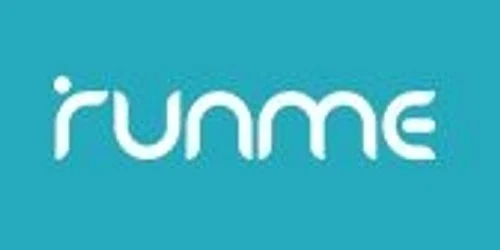
Rune Apps Puzzle Box Solver
Adding other assets, like images or fonts, directly to the component is not possible out-of-the-box, but there are ways to do it.
To Recap...
There are command line tools to scaffold a new project for both React and Angular.
For React, there's create-react-app, which you will run only to create the application. But under the hood, your package.json
uses react-scripts when you run, test or build your application. While create-react-app is a convenient way to bootstrap your application, you are not required to use it: There are other ways to get started with React, too.
Rune App Jad
Angular, on the other hand, comes with the Angular CLI, and it is highly recommended that you use it. You will not only run it to create your application, but also later on during development.
Runme App
Both tools scaffold an application for you. They provide ways to add static assets and per-component style sheets. The React project also comes with a webpack configuration that allows you to add other assets directly to your components, while in Angular, those other assets should go into the static assets folder.
Runme.app Download
Do you want to be notified when I publish new content? Follow @dtanzer on Twitter! Or would you like more content like this in your inbox from time to time? Subscribe here: